C Neural Network Library: Genann
Summary
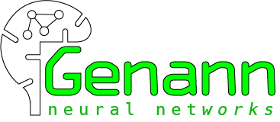
Genann is a minimal, well-tested open-source library implementing feedfordward artificial neural networks (ANN) in C. It's entirely contained in a single C source file and header file, so it's easy to add to your projects. Genann has a focus on being easy to use but is also very extensible.
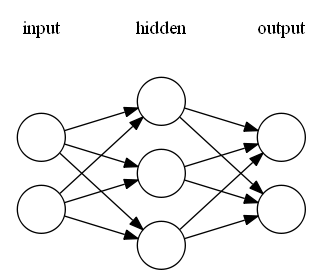
Code Sample
#include "genann.h"
/* Not shown, loading your training and test data. */
double **training_data_input, **training_data_output, **test_data_input;
/* New network with 2 inputs,
* 1 hidden layer of 3 neurons each,
* and 2 outputs. */
genann *ann = genann_init(2, 1, 3, 2);
/* Learn on the training set with backpropagation. */
for (i = 0; i < 300; ++i) {
for (j = 0; j < 100; ++j)
genann_train(ann, training_data_input[j], training_data_output[j], 0.1);
}
/* Run the network and see what it predicts. */
double const *prediction = genann_run(ann, test_data_input[0]);
printf("Output for the first test data point is: %f, %f\n", prediction[0], prediction[1]);
genann_free(ann);
Features
- ANSI C with no dependencies.
- Contained in a single source code and header file.
- Simple, fast, thread-safe, easily extendible.
- Implements backpropagation training.
- Compatible with alternative training methods (classic optimization, genetic algorithms, etc)
- Includes examples and test suite.
- Released under the zlib license - free for nearly any use.